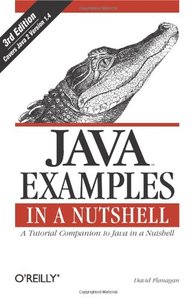
Java Examples in a Nutshell, 3/e
內容描述
The author of the best-selling Java in a Nutshell has created an
entire book of real-world Java programming examples that you can learn from. If
you learn best "by example," this is the book for you. This third
edition covers Java 1.4 and contains 193 complete, practical examples: over
21,900 lines of densely commented, professionally written Java code, covering 20
distinct client-side and server-side APIs. It includes new chapters on the Java
Sound API and the New I/O API. The chapters on XML and servlets have been
rewritten to cover the latest versions of the specifications and to demonstrate
best practices for Java 1.4. New and updated examples throughout the book
demonstrate many other new Java features and APIs. Java Examples in a
Nutshell is a companion volume to Java in a Nutshell, Java
Foundation Classes in a Nutshell, and Java Enterprise in a Nutshell.
It picks up where those quick references leave off, providing a wealth of
examples for both novices and experts. This book doesn't hold your hand; it
simply delivers well-commented working examples with succinct explanations to
help you learn and explore Java and its APIs. Java Examples in a
Nutshell contains examples that demonstrate:
Core APIs, including I/O, New I/O, threads, networking, security,
serialization, and reflection
Desktop APIs, highlighting Swing GUIs, Java 2D graphics, preferences,
printing, drag-and-drop, JavaBeans, applets, and sound
Enterprise APIs, including JDBC (database access), JAXP (XML parsing and
transformation), Servlets 2.4, JSP 2.0 (JavaServer Pages), and RMI
The book begins with introductory examples demonstrating structured and
object-oriented programming techniques for new Java programmers. A special index
at the end of the book makes it easy to look up examples that use a particular
Java class or accomplish a desired task. In between, each chapter includes
exercises that challenge readers and suggest further avenues for exploration
Table of ContentsPreface
I. Learning Java
Java Basics
Hello World
FizzBuzz
The Fibonacci Series
Using Command-Line Arguments
Echo in Reverse
FizzBuzz Switched
Computing Factorials
Recursive Factorials
Caching Factorials
Computing Big Factorials
Handling Exceptions
Interactive Input
Using a StringBuffer
Sorting Numbers
Computing PrimesObjects, Classes, and Interfaces
A Rectangle Class
Testing the Rect Class
A Rect Subclass
Another Subclass
Complex Numbers
Computing Statistics
An Integer List
Tokenizing Text
II. Core Java APIs
Input/Output
Files and Streams
Working with Files
Copying File Contents
Reading and Displaying Text Files
Listing Directory and File Information
Compressing Files and Directories
Filtering Character Streams
Tokenizing a Character Stream
Random Access to FilesThreads
Thread Basics
Thread-Safe Classes
Threads and Thread Groups
Deadlock
TimersNetworking
Downloading the Contents of a URL
Using a URLConnection
Sending Email Through a URLConnection
A Simple Network Client
A Generic Client
An HTTP Client
A POP Client
A Simple Web Server
A Proxy Server
A Generic Multithreaded Server
Sending Datagrams
Receiving DatagramsNew I/O
Locking Files
Copying Files
Regular Expressions and Character Decoding
File Copying with Buffers
Advanced Byte-to-Character Conversion
Tokenizing Byte Buffers
A Simple HTTP Client
The Daytime Service
A Multiplexed Server
A Multiplexed Network ClientSecurity and Cryptography
Running Untrusted Code
Loading Untrusted Code
Message Digests and Digital Signatures
CryptographyInternationalization
A Word About Locales
Unicode
Character Encodings
Handling Local Customs
Localizing User-Visible Messages
Formatted MessagesReflection
Obtaining Class and Member Information
Invoking a Named Method
Proxy ObjectsObject Serialization
Simple Serialization
Custom Serialization
Externalizable Classes
Serialization and Class Versioning
III. Desktop Java APIs
Graphical User Interfaces
Components
Containers
Layout Management
Event Handling
A Complete GUI
Actions and Reflection
Custom Dialogs
An Error Handler Dialog
Displaying Tables
Displaying Trees
A Simple Web Browser
Describing GUIs with Properties
Themes and the Metal Look-and-Feel
Look-and-Feel Preferences
The ShowBean ProgramGraphics
Graphics Before Java 1.2
The Java 2D API
Drawing and Filling Shapes
Transforms
Line Styles with BasicStroke
Stroking Lines
Filling Shapes with Paint
Antialiasing
Combining Colors with AlphaComposite
Image Processing
Image I/O
Custom Shapes
Custom Strokes
Custom Paint
Advanced Animation
Displaying Graphics ExamplesPrinting
Printing with the Java 1.1 API
Printing with the Java 1.2 API
Printing with the Java 1.4 API
Printing Multipage Text Documents
Advanced Printing with Java 1.4Data Transfer
Simple Swing Data Transfer
A Clock with Drag and Copy Support
Data Transfer Architecture
Dropping Multiple Datatypes
A Transferable Shape
Custom Data TransferJavaBeans
Bean Basics
A Simple Bean
A More Complex Bean
Custom Events
Specifying Bean Information
Defining a Simple Property Editor
Defining a Complex Property Editor
Defining a Bean Customizer
Manipulating BeansApplets
Introduction to Applets
A First Applet
A Clock Applet
A Timer Applet
Applets and the Java 1.0 Event ModelSound
Ringing the Bell
Swing Aural Cues
Playing Sounds with AudioClip
Playing Sounds with javax.sound
Streaming Sounds with javax.sound
Synthesizing a MIDI Sequence
Real-Time MIDI Sounds
IV. Enterprise Java APIs
Database Access with SQL
Accessing a Database
Using Database Metadata
Building a Database
Using the API Database
Atomic TransactionsXML
Parsing with JAXP and SAX
Parsing and Manipulating with JAXP and DOM
Transforming XML with XSLT
An XML Pull ParserServlets and JavaServer Pages
Servlet Setup
A Hello World Servlet
Another Simple Servlet
Servlet Initialization and Persistence: A Counter Servlet
Hello JSP
Hello JSP2
Hello XML
The MVC Paradigm for Web Applications
ListManager Model Classes
ListManager Controller
ListManager Views
Custom Tags in JSP 2.0
Packaging a Web ApplicationRemote Method Invocation
Remote Banking
A Bank Server
A Persistent Bank Server
A Multiuser Domain
Remote MUD Interfaces
The MUD Server
The MudPlace Class
The MudPerson Class
A MUD Client
Advanced RMIExample Index
Index